How to write to a text file in Python
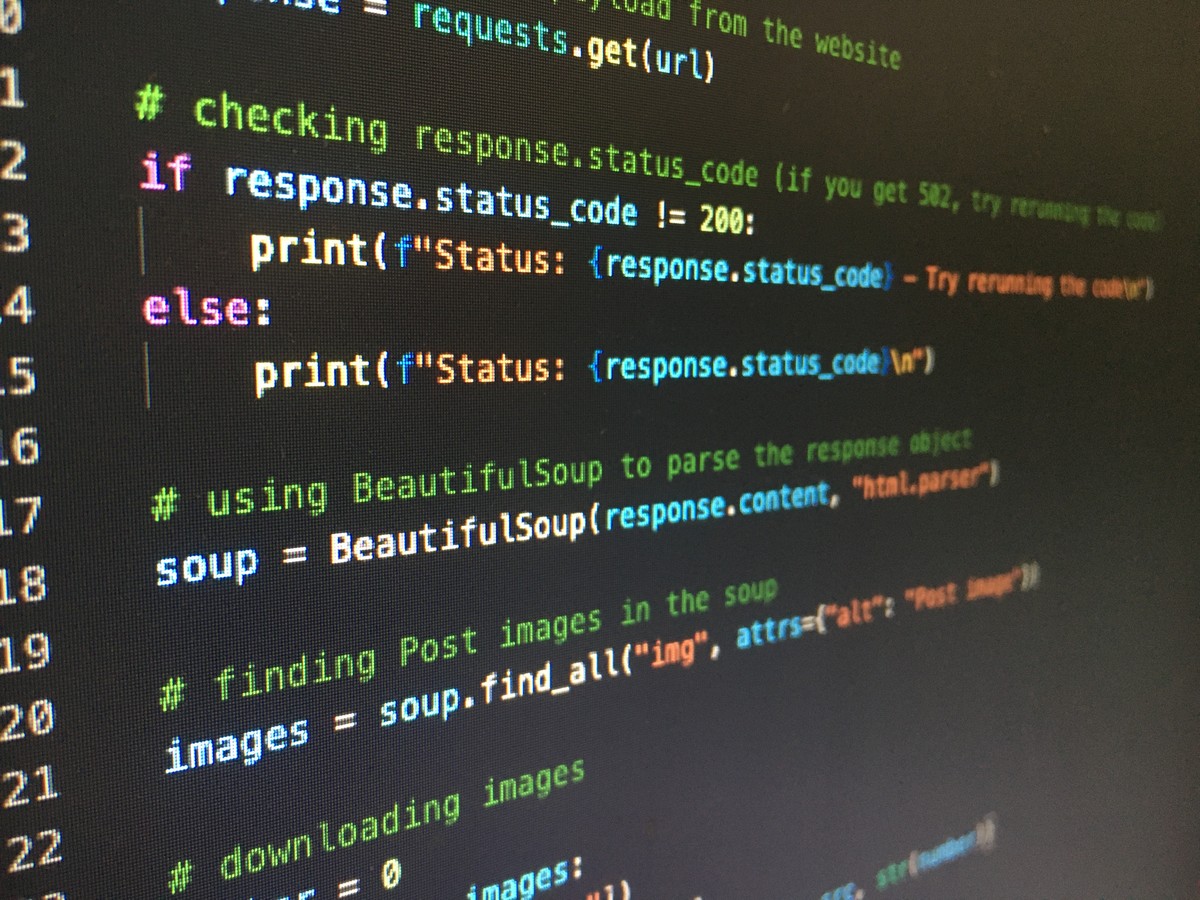
When it comes to working with text files in Python, understanding how to write data to them is an essential skill. In this article, we will explore the process of writing to text files using the Python programming language.
By following a few simple steps, you'll be able to create and modify text files effortlessly. So, let's dive in and learn the basics of writing text files in Python.
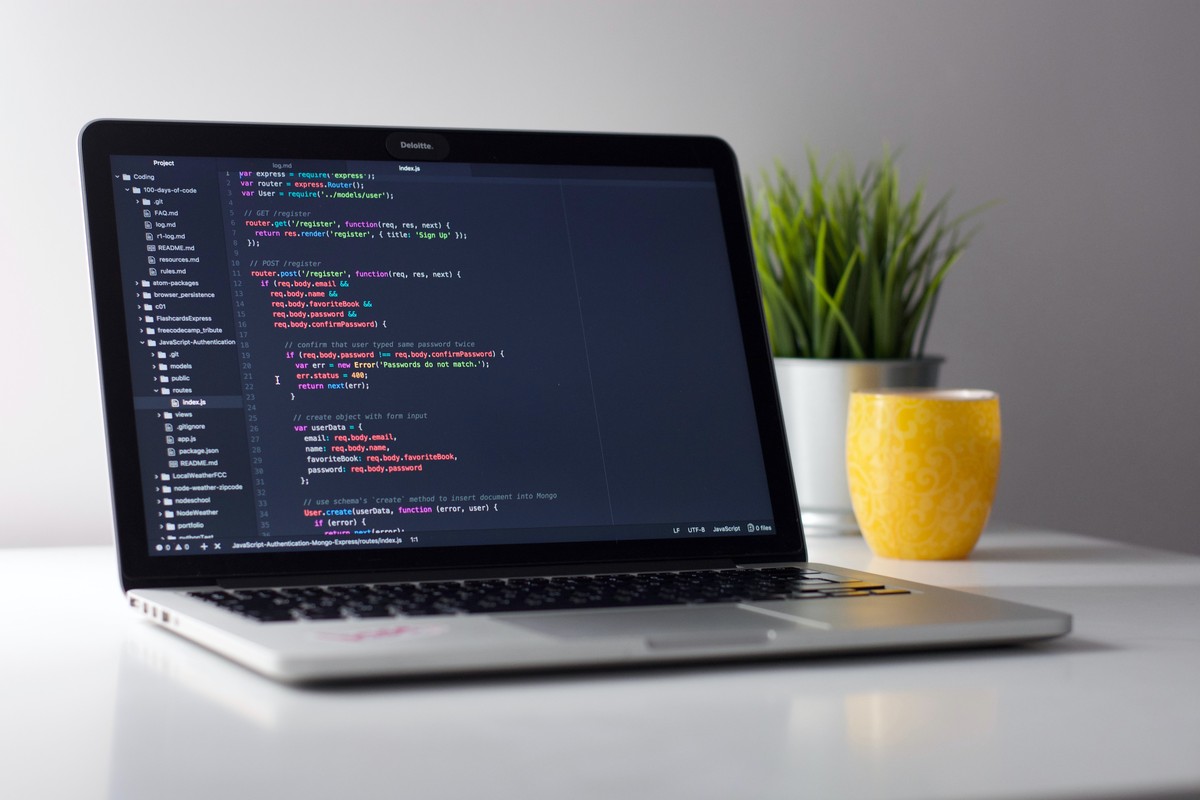
How to write to a text file in Python
To begin writing to a text file, the first step is to open the file using the open() function. This function requires two arguments: the path to the file you want to open and the mode in which you want to open it.
For writing purposes, you need to specify the mode as 'w'. If the specified file doesn't exist, Python will create a new file with the given name.
Let's take a look at an example:
with open("example.txt", "w") as f:
# Code for writing to the file goes here
In the above code, we open a file named "example.txt" in write mode by passing 'w' as the mode parameter to the open() function. The with statement ensures that the file is automatically closed once we're done writing.
Writing to text file
Once the file is open, we can proceed to write content into it. To accomplish this, we use the write() method, which takes a string as an argument and writes it to the file.
For instance, let's write the string "Hello world!" to our "example.txt" file:
with open("example.txt", "w") as f:
f.write("Hello world!")
In the above code, the write() method is used to add the "Hello world!" string to the file. It's important to note that each call to write() will append the provided string to the existing content of the file.
Closing the file
To finalize the writing process and ensure that all the changes are saved, it's crucial to close the text file. You can accomplish this by invoking the close() method on the file object.
However, a more convenient approach is to use the with statement, as shown in the previous examples. The with statement automatically takes care of closing the file for you once you exit its scope.
With these three simple steps, you now have a solid understanding of how to write to text files in Python. Remember to open the file in write mode ('w'), use the write() method to add content, and close the file either explicitly with close() or implicitly with the with statement.
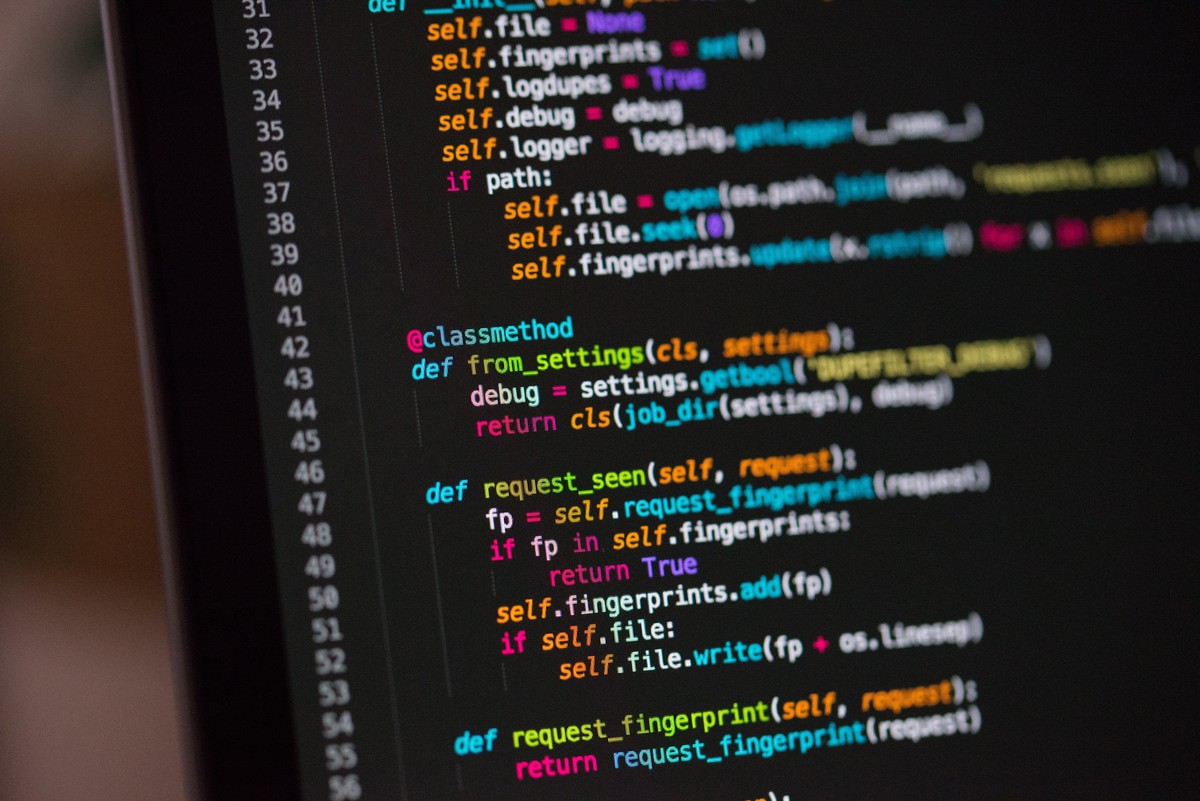
One last method
In addition to the write() method, Python provides the writelines() method, which allows you to write multiple strings at once. This method takes an iterable object, such as a list, and writes each element as a separate line in the file.
Here's an example:
words = ["This", "is", "a", "test"]
with open("example.txt", "w") as f:
f.writelines(words)
In the code above, the writelines() method writes each word from the words list to the file, resulting in each word appearing on a separate line.
Congratulations! You have successfully learned how to write to text files in Python. Now you can confidently open, write, and close text files using the open(), write(), and close() methods, or by leveraging the convenience of the with statement.
Practice these techniques, and you'll be well-equipped to handle various file-writing tasks in your Python projects. Keep coding and enjoy exploring the vast possibilities of Python!
Advertisement
This is a high-level (pun intended) overview of how mainframes get hacked. :-P
bro who are you competing with? Who will go here for basic python??